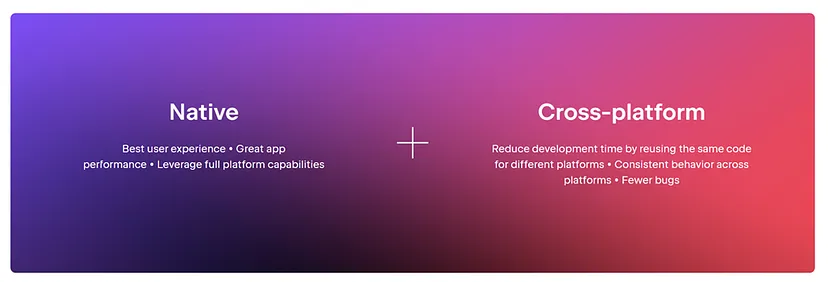
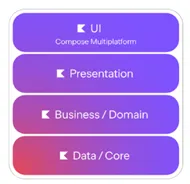
a. Kotlin Multiplatform facilitates the sharing of common code across all platforms utilized in your project.
b. This approach involves retaining native code for the UI and certain key components, ensuring a balance between shared logic and platform-specific elements.
c. Refer to the chart below for a visual representation of how Kotlin Multiplatform operates to enable efficient code sharing between platforms
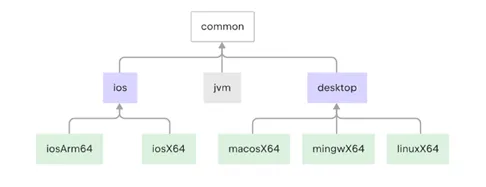
a. For Android development, ensure you have the standard Android development environment set up, including Android Studio, Java, and Kotlin.
b. For iOS, installation of Xcode on a Mac with macOS is required, along with Java.
c. Install the Kotlin Multiplatform Mobile plugin on Android Studio through the plugins section. Additional details can be found here.
a. In Android Studio, choose “New Kotlin Multiplatform App” from the new project section. This will generate a new Android/iOS project with Kotlin Multiplatform dependencies ready.
b. The project structure includes three modules:
- shared: A Kotlin module containing common logic shared between Android and iOS applications.
- androidApp: A Kotlin module building into an Android application.
- iosApp: An Xcode project building into an iOS application, using the shared module as an iOS framework.
a. The shared module consists of three source sets: androidMain, commonMain, and iosMain. Different source sets target different platforms, utilizing Kotlin/JVM for androidMain and Kotlin/Native for iosMain.
b. The expect/actual mechanism binds platforms with KMP code. Shared interfaces are defined in the shared module, and platform-specific implementations are provided in each platform module.
a. Open Greeting.kt in the commonMain package. The greet() function and the Platform instance generated from getPlatform() method are defined here.
b. The Platform interface in Platform.kt includes a variable name and a function getPlatform(). The expect keyword precedes getPlatform(), signifying the Expect/Actual mechanism for KMP.
c. Platform-specific implementations are found in Platform.Android.kt and Platform.Ios.kt, returning instances of AndroidPlatform and IOSPlatform respectively.
a. Navigate to MainActivity in the androidApp module, where the Greeting class is imported from the shared module.
b. Call Greeting().greet() to execute shared logic within the Android application.
a. Similarly, in the iOS module (iosApp), integrate the shared module as a dependency.
b. Open ContentView and import the shared module. Call Greeting().greet() to execute shared logic within the iOS application.
Key Points to Note from the Code:
- Expect/Actual Mechanism: The use of expect/actual is crucial for binding Kotlin Multiplatform (KMP) with native projects. This mechanism allows developers to define shared interfaces in the shared module and provide platform-specific implementations in each platform module, facilitating seamless cross-platform integration.
- androidApp Module: The androidApp module closely resembles a typical Android application codebase. Developers can leverage standard Android code practices within this module, making it familiar for Android developers transitioning to Kotlin Multiplatform.
- iosApp Module:The iosApp module represents a standard iOS project with the shared module as a dependency. When opened in Xcode, it appears as a regular iOS project, providing developers the freedom to incorporate and code any iOS-specific functionalities within this module. This allows for a natural and platform-specific development experience in the iOS environment.
Understanding these key aspects is essential for effectively utilizing Kotlin Multiplatform in cross-platform development. The combination of the Expect/Actual mechanism and platform-specific modules ensures a smooth integration process, allowing developers to harness the strengths of each platform while sharing common logic efficiently.
This should be enough knowledge to get started with simple code sharing application.
REST API integration is a fundamental aspect of sharing logic between mobile applications, and Kotlin Multiplatform (KMP) provides an efficient way to achieve this. When working with KMP, using standard libraries like Retrofit for Android or Alamofire for iOS may not be feasible, as Retrofit relies on the JVM, and Alamofire depends on core iOS frameworks.
To address this, KMP recommends using Ktor for implementing REST API-related logic. Ktor is a flexible and asynchronous Kotlin web framework that works seamlessly with Kotlin Multiplatform projects. Below are the steps to implement REST API logic with Ktor.
- Ktor Integration:Integrate Ktor into your Kotlin Multiplatform project. Ensure that you have the necessary dependencies and configurations set up. You can refer to the provided link for detailed instructions on implementing REST API logic with Ktor.
- Platform-Independent Code:Write platform-independent code in the shared module to handle the common logic related to REST API interactions. This may include defining data models, API endpoints, and logic for making network requests.
- Platform-Specific Implementations:Utilize the Expect/Actual mechanism to provide platform-specific implementations for making HTTP requests. Define the expected behavior in the shared module and implement the actual HTTP request logic in the platform-specific modules for Android and iOS.
- Ktor Features:Leverage Ktor’s features such as the HttpClient for making HTTP requests, routing for defining API endpoints, and serialization for handling data formats like JSON. Ktor’s asynchronous nature aligns well with Kotlin Multiplatform’s goal of providing efficient cross-platform development.
By following these steps and integrating Ktor, developers can seamlessly share REST API-related logic across Android and iOS platforms within a Kotlin Multiplatform project. This approach ensures a consistent and unified codebase while catering to the specific requirements of each platform. Refer to the provided link for more detailed guidance on implementing REST API logic with Ktor in Kotlin Multiplatform.
more at :- https://ktor.io/docs/getting-started-ktor-client-multiplatform-mobile.html
In Kotlin Multiplatform projects, establishing a common database for offline applications is crucial, and SQLDelight emerges as a robust option for creating a shared module with a database. Here’s a concise overview and benefits of using SQLDelight:
- Platform-Specific Drivers: SQLDelight simplifies the handling of platform-specific database implementations. Under the hood, it manages drivers for each platform, ensuring optimal performance and compatibility. This abstraction shields developers from dealing with intricate platform-specific details, streamlining the database integration process.
- Type-Safe Queries: One of the standout features of SQLDelight is its support for type-safe queries. By generating SQL queries at compile-time, it eliminates runtime surprises and guarantees type safety. This proactive approach not only reduces errors but also enhances code maintainability, providing developers with peace of mind.
- Raw SQL for Flexibility: SQLDelight encourages developers to embrace the power and flexibility of raw SQL. This approach allows for the execution of complex queries and interactions with the database without abstracting away the SQL language. Developers can leverage the full potential of SQL, staying in control of their database interactions.
- Integration with Kotlin Features:SQLDelight seamlessly integrates with Kotlin’s core features, including coroutines, flow, and multiplatform architecture. This ensures a smooth development experience, allowing developers to leverage the strengths of Kotlin while working with the database. The integration with coroutines and flow facilitates asynchronous and reactive programming paradigms.
- Efficient Codebase Sharing:SQLDelight significantly contributes to codebase reduction by enabling the sharing of a substantial portion of database logic across multiple platforms. This reduction in code duplication amplifies development efficiency, making it an effective solution for projects targeting diverse platforms.
By adopting SQLDelight, Kotlin Multiplatform developers can establish a common and efficient database solution for offline applications. The platform-specific abstraction, type-safe queries, and seamless integration with Kotlin features contribute to a streamlined and productive development experience. This approach not only simplifies database management but also enhances the overall maintainability and reliability of Kotlin Multiplatform projects.
sample can be found here:- https://www.jetbrains.com/help/kotlin-multiplatform-dev/multiplatform-ktor-sqldelight.html#configure-sqldelight-and-implement-cache-logic
- Challenge: Writing code for multiple platforms, such as web, mobile, and desktop, using different technologies can lead to significant code redundancy and increased development effort.
- Solution: KMP facilitates code sharing across platforms, effectively reducing redundancy and streamlining development. Developers can focus on writing core logic once and reuse it across various platforms, promoting code efficiency.
- Challenge: Duplicating code logic and business rules for multiple platforms can introduce human errors and inconsistencies. Different platform limitations and varying databases may result in inconsistent user experiences.
- Solution: KMP addresses this challenge by allowing developers to share a common codebase while building separate apps for each platform. This ensures a consistent user experience, as the shared logic remains uniform across diverse platforms.
- Challenge: Using certain hybrid or cross-platform technologies may restrict access to native features, and updating the app to align with the latest changes on native platforms can be challenging.
- Solution: KMP enables developers to keep the core app logic on the native platform, eliminating the limitations associated with hybrid platforms. With KMP, developers can leverage all native features while minimizing code redundancy.
- Challenge: Adopting new cross-platform frameworks often involves learning new languages, tools, and paradigms, which can contribute to a steep learning curve for developers.
- Solution: KMP leverages the existing Kotlin language and ecosystem, making it familiar and easier to learn for Kotlin developers. This reduces the learning curve and accelerates the development process. Additionally, allowing developers to keep the UI part in native platforms enables a smoother adoption process compared to other cross-platform solutions.